Mastering Clean Code: Best Practices for Error-free, Readable, and Lightweight C# Programming
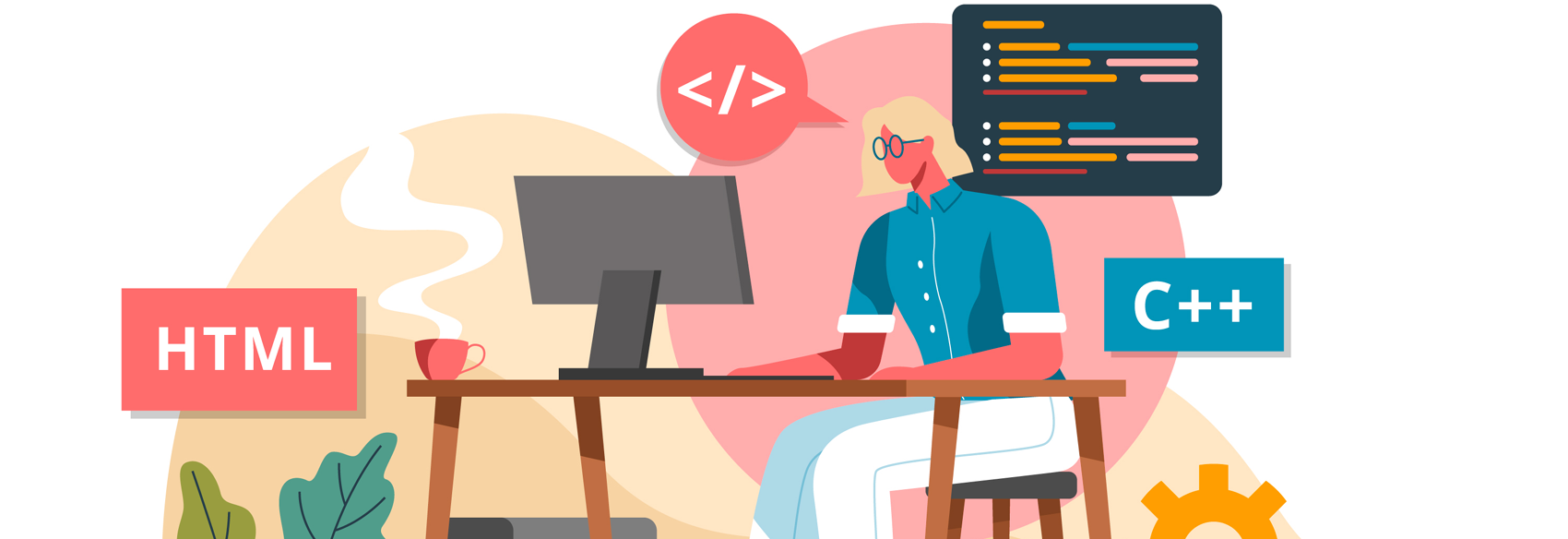
Clean code is the hallmark of a skilled software developer. It not only improves code readability but also reduces errors and makes maintenance and scalability easier. In this blog post, we will explore some best coding practices, error handling techniques, and code optimization tips to help you write clean, efficient, and reliable C# code.
1. Meaningful and Consistent Naming Conventions:
One of the fundamental principles of writing clean code is to use meaningful names for variables, functions, classes, and other entities. Ensure that your code follows a consistent naming convention (e.g., camel case or Pascal case) throughout the project. Meaningful names make code more readable, self-documenting, and provide context to fellow developers. Consistency is an essential aspect of clean code. Adopt a consistent naming convention for variables, functions, and classes. Use descriptive names that convey their purpose, making it easier for others to understand and maintain the code.
// C#
// Example of meaningful variable names
int studentAge = 20;
string studentName = "John Doe";
// Example of meaningful function names
public bool IsAdult(int age)
{
return age >= 18;
}
2. Proper Indentation and Formatting:
Consistent indentation and code formatting greatly enhance code readability. Use proper spacing and line breaks to organize your code logically. Avoid long lines of code and wrap them to fit within a reasonable length (80-120 characters) for better legibility.
// C#
// Example of properly indented and formatted code
public void CalculateSum(int a, int b)
{
int sum = a + b;
Console.WriteLine("The sum of {0} and {1} is {2}", a, b, sum);
}
3. Maintain Short and Focused Methods:
To promote code readability and reusability, keep your methods short, focused, and following the Single Responsibility Principle(SRP). Each method should have a clear purpose and do only one thing. Breaking down complex logic into smaller, modular methods not only aids comprehension but also simplifies testing and debugging. Divide your code into smaller, more manageable modules that each have a single responsibility. This approach allowing for code reuse, easier testing, and improved readability. Avoid long and monolithic functions or classes that become hard to comprehend and maintain.
// C#
// Example of breaking down complex logic into smaller methods
public int CalculateSum(int a, int b)
{
return a + b;
}
public void DisplaySum(int sum)
{
Console.WriteLine("The sum is: " + sum);
}
// Usage
int result = CalculateSum(3, 4);
DisplaySum(result);
4. Effective Error Handling:
Clean code should handle errors gracefully and provide helpful messages for debugging and troubleshooting. Utilize exception handling techniques such as try-catch-finally blocks to catch and handle exceptions appropriately. Avoid using broad catch-all exceptions; instead, catch specific exceptions that you can handle explicitly.
// C#
// Example of proper error handling
try
{
int[] numbers = new int[] { 1, 2, 3 };
int result = numbers[4]; // accessing an out-of-bounds index
}
catch (IndexOutOfRangeException ex)
{
Console.WriteLine("An error occurred: " + ex.Message);
}
5. Optimize Code for Performance:
Clean code also means optimizing performance by reducing unnecessary computations, memory usage, and improving code efficiency. Utilize built-in data structures and algorithms whenever possible and avoid reinventing the wheel.
// C#
// Example of using built-in C# features for efficient code
List<int> numbers = new List<int>() { 1, 2, 3 };
foreach (int number in numbers)
{
Console.WriteLine(number);
}
6. Minimize Dependencies:
Reduce coupling and dependencies between different parts of your code. Code that is tightly coupled becomes challenging to understand and maintain. Use dependency injection and interfaces to decouple components, promoting code reuse and testability.
7. Code Refactoring and Simplicity:
Refactor your code frequently to eliminate duplication, improve performance, and simplify the implementation. Don't be afraid to rewrite and optimize code if necessary. Simplicity should always be prioritized over complexity to enhance readability.
Conclusion:
Writing clean code in C# goes beyond adhering to a set of guidelines. It requires a mindset that prioritizes readability, error handling, and code optimization. By following best practices, using meaningful naming conventions, maintaining proper indentation, and employing efficient error handling, you can ensure your code is clean, easy to understand, and performs optimally. Remember, clean code benefits not only you but also the other developers who work on the project. So, strive for clean code and become a better programmer.